Get Started with the Twilio API for WhatsApp
The Twilio API for WhatsApp is the quickest way to integrate two-way messaging on WhatsApp into any web application including Salesforce using 6 popular web languages (Python, C#/.NET, PHP, Java, Node.js, Ruby). In the below Quickstart we will see how to do this using the Twilio Sandbox for WhatsApp, Python, the Twilio Python helper library, and the Flask web framework.
For a quick introduction to Twilio and Demo on Salesforce WhatsApp integration refer our previous article here
Twilio API for WhatsApp Python Quickstart
Sign up for Twilio and activate the Sandbox
Before you can send a WhatsApp message from your web language, you'll need to sign up for a Twilio account or sign into your existing account and activate the Twilio Sandbox for WhatsApp. It allows you to prototype with WhatsApp immediately using a shared phone number, without waiting for a dedicated number to be approved by WhatsApp.
Gather your Twilio account information:
Once you signup for Twilio, it will launch Twilio Console. Make a note of following details from Console which will be used in the Heroku app.
Opt-in to the Sandbox
Once you join the sandbox by sending the message, you will receive a confirmation message as shown below:
Send Messages from Salesforce to WhatsApp
In our demo case whenever an executive adds a comment to the Case or when a new case is created the corresponding trigger handler makes a callout to the Heroku app that has the Twilio Python wrapper code hosted.
Below is the Apex code making callout to Heroku
public class CaseCommentTriggerHandler{
@future(callout=true)
public static void createCaseComment(List<Id> csIds){
List<CaseComment> csList = new List<CaseComment>();
if(csIds != null && csIds.size() > 0){
csList = [SELECT id,
CommentBody,
Parent.CaseNumber,
Parent.Subject,
Parent.Description,
Parent.ContactPhone
FROM
CaseComment WHERE Id IN :csIds];
}
Http h = new Http();
HttpResponse response = new Httpresponse();
HttpRequest req = new HttpRequest();
req.setMethod('GET');
String endpoint = '';
if(csList != null && csList.size () > 0){
String caseComments = EncodingUtil.URLENCODE('Salesforce Case '+csList[0].Parent.CaseNumber+' has been updated for you with the comments: '+csList[0].CommentBody,'UTF-8');
endpoint =''+csList[0].Parent.ContactPhone+'&msg='+caseComments;
}
req.setEndpoint(endpoint);
response = h.send(req);
}
}
And below is the Twilio Python wrapper code and following are the Auth parameters configured in Heroku in Config Vars
from flask import Flask
from flask import request
import os
from twilio.rest import Client
app = Flask(__name__)
@app.route('/')
def twilio_heroku_app():
account_sid = os.environ.get('account_sid', None)
auth_token = os.environ.get('auth_token', None)
sandbox_number = os.environ.get('sandbox_number', None)
to_number = request.args.get('to')
msg_body = request.args.get('msg')
client = Client(account_sid, auth_token)
message = client.messages.create(
body=msg_body,
from_='whatsapp:'+sandbox_number,
to='whatsapp:+91'+to_number
)
return message.sid
if __name__ == '__main__':
app.run()
Capture Replies from WhatsApp in Salesforce
When a customer replies to your WhatsApp Sandbox number, the same can be captured in Salesforce by configuring a web service in Salesforce with Twilio Webhook as shown below. The Salesforce web service must accept POST request and will have the business logic to perform desired actions like logging cases in Salesforce etc.
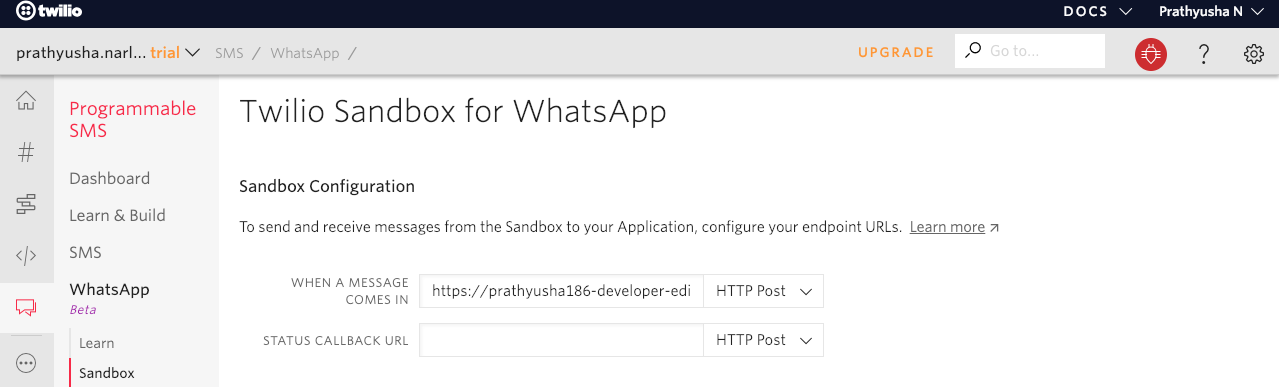
The web service in Salesforce will receive the Message text, the From number, the To number and other details in the body of the request that can be used to create a Case or add comment to an open case for the existing customer (that can be identified from the Contact and/or Account created with the From number) upon receiving a message in WhatsApp.
In subsequent articles I will explore the other communication channels available with Twilio and integrate the same with Salesforce.